Mastering Data Structures and Algorithms: A Comprehensive Guide for Beginners
Monday 06, 2024
//8 mins, 1611 words
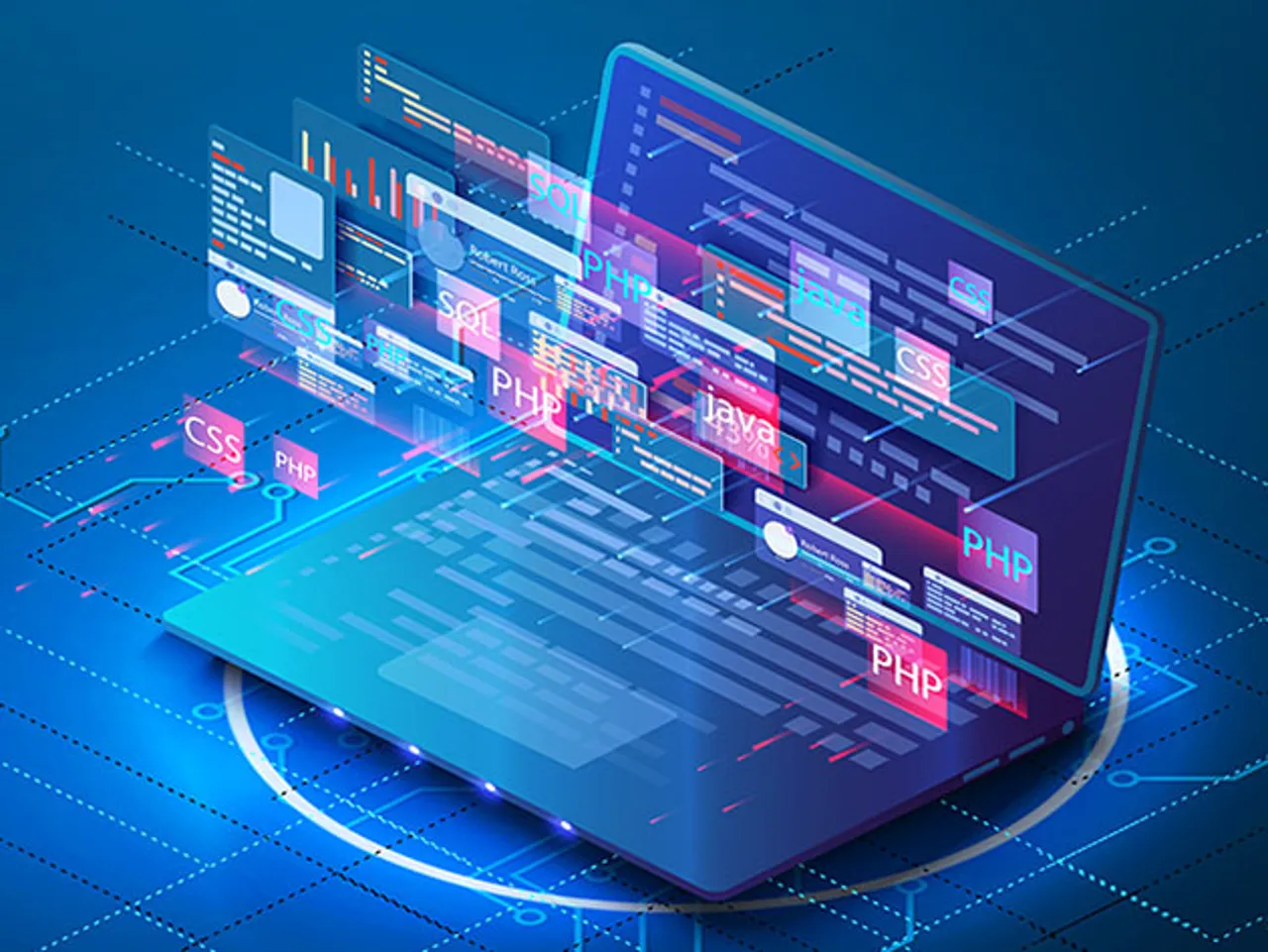
Mastering Data Structures and Algorithms: A Comprehensive Guide for Beginners
Introduction
In programming, understanding data structures and algorithms is crucial for building efficient and scalable software solutions. Data structures are ways of organizing and storing data, while algorithms are step-by-step procedures for solving problems or performing computations.
Mastering these concepts improves your problem-solving skills and enhances your ability to write clean and optimized code. Now, as we delve deeper into mastering C programming, it's time to explore advanced topics that will further enhance your skills and understanding.
Mastering data structures and algorithms is a crucial aspect of becoming proficient in programming. These concepts build upon the basics we've already covered and provide you with powerful tools to solve complex problems efficiently.
Here are some more topics that will help you understand C programming.
Arrays
Arrays are a fundamental data structure that stores elements of the same type in contiguous memory locations. They provide fast access to elements using an index.
Example in C++:
#include
using namespace std;
int main() {
// Declaring an array of integers
int arr[5] = {1, 2, 3, 4, 5};
// Accessing elements of the array
cout << "Elements of the array: ";
for (int i = 0; i < 5; ++i) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
Linked Lists
Linked lists are linear data structures consisting of nodes, where each node contains a data element and a pointer to the next node in the sequence.
Example in C++:
#include
using namespace std;
// Define the structure for a node in the linked list
struct Node {
int data;
Node* next;
};
int main() {
// Creating nodes for a singly linked list
Node* head = new Node();
head->data = 1;
Node* second = new Node();
second->data = 2;
head->next = second;
second->next = NULL;
// Traversing the linked list
Node* current = head;
while (current != NULL) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
return 0;
}
Stacks and Queues
Stacks and queues are abstract data types that allow the insertion and removal of elements following specific rules. Stacks follow the Last-In-First-Out (LIFO) principle, while queues follow the First-In-First-Out (FIFO) principle.
Example in C++ (Stack)
#include
#include
using namespace std;
int main() {
stack s;
// Pushing elements onto the stack
s.push(1);
s.push(2);
s.push(3);
// Popping elements from the stack
while (!s.empty()) {
cout << s.top() << " ";
s.pop();
}
cout << endl;
return 0;
}
Example in C++ (Queue)
#include
#include
using namespace std;
int main() {
queue q;
// Enqueuing elements into the queue
q.push(1);
q.push(2);
q.push(3);
// Dequeuing elements from the queue
while (!q.empty()) {
cout << q.front() << " ";
q.pop();
}
cout << endl;
return 0;
}
Trees
Trees are hierarchical data structures composed of nodes, where each node has a value and a list of references to its child nodes. Common types of trees include binary trees, binary search trees, and balanced trees.
Example in C++ (Binary Tree):
#include
using namespace std;
// Define the structure for a node in the binary tree
struct Node {
int data;
Node* left;
Node* right;
};
// Function to create a new node
Node* createNode(int data) {
Node* newNode = new Node();
newNode->data = data;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
int main() {
// Create a binary tree
Node* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
// Traverse the binary tree (preorder traversal)
cout << "Preorder traversal of the binary tree: ";
preorder(root);
cout << endl;
return 0;
}
Graphs
Graphs are collections of vertices (nodes) connected by edges. They can be represented using adjacency lists or adjacency matrices. Graph traversal algorithms, such as depth-first search (DFS) and breadth-first search (BFS), are used to visit and explore the vertices of a graph.
Example in C++ (Graph Traversal - DFS):
#include
#include
#include
using namespace std;
// Function to perform a depth-first search (DFS) on a graph
void DFS(vector>& graph, int start) {
stack s;
vector visited(graph.size(), false);
s.push(start);
while (!s.empty()) {
int vertex = s.top();
s.pop();
if (!visited[vertex]) {
cout << vertex << " ";
visited[vertex] = true;
for (int neighbor : graph[vertex]) {
if (!visited[neighbor]) {
s.push(neighbor);
}
}
}
}
}
int main() {
// Example graph represented using an adjacency list
vector> graph = {
{1, 2}, // Node 0 is connected to nodes 1 and 2
{0, 3, 4}, // Node 1 is connected to nodes 0, 3, and 4
{0, 5}, // Node 2 is connected to nodes 0 and 5
{1}, // Node 3 is connected to Node 1
{1}, // Node 4 is connected to Node 1
{2} // Node 5 is connected to Node 2
};
cout << "Depth-First Search (DFS) traversal of the graph: ";
DFS(graph, 0); // Start DFS traversal from node 0
cout << endl;
return 0;
}
Sorting Algorithms
Sorting algorithms are used to arrange elements in a specific order, such as numerical or lexicographical order. Common sorting algorithms include bubble sort, insertion sort, selection sort, merge sort, and quicksort.
Example in C++ (Bubble Sort):
#include
using namespace std;
// Function to perform bubble sort
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
int arr[] = {64, 25, 12, 22, 11};
int n = sizeof(arr) / sizeof(arr[0]);
cout << "Array before sorting: ";
for (int i = 0; i < n; ++i) {
cout << arr[i] << " ";
}
cout << endl;
bubbleSort(arr, n);
cout << "Array after sorting: ";
for (int i = 0; i < n; ++i) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
Searching Algorithms
Searching algorithms are used to find a particular element within a collection of data. Common searching algorithms include linear search and binary search.
Example in C++ (Binary Search):
#include
using namespace std;
// Function to perform binary search on a sorted array
int binarySearch(int arr[], int left, int right, int target) {
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Element not found
}
int main() {
int arr[] = {2, 5, 7, 12, 18, 23, 30};
int n = sizeof(arr) / sizeof(arr[0]);
int target = 18;
int index = binarySearch(arr, 0, n - 1, target);
if (index != -1) {
cout << "Element found at index " << index << endl;
} else {
cout << "Element not found." << endl;
}
return 0;
}
Recursion
Recursion is a programming technique where a function calls itself to solve a problem. It involves breaking down a problem into smaller, similar subproblems until a base case is reached.
Example in C++ (Factorial Calculation):
#include
using namespace std;
// Function to calculate the factorial of a non-negative integer
int factorial(int n) {
if (n == 0 || n == 1) {
return 1; // Base case: factorial of 0 and 1 is 1
} else {
return n * factorial(n - 1); // Recursive case
}
}
int main() {
int n = 5;
int result = factorial(n);
cout << "Factorial of " << n << " is " << result << endl;
return 0;
}
In this example, the factorial function calls itself recursively to calculate the factorial of a given non-negative integer.
In "The Ultimate Beginner's Guide to C Programming," we embarked on a journey to introduce you to the fundamental concepts of C programming. We covered essential topics such as variables, control flow statements, and functions, laying a solid foundation for your programming journey.